API Rate Limiting: Principles and Best Practices
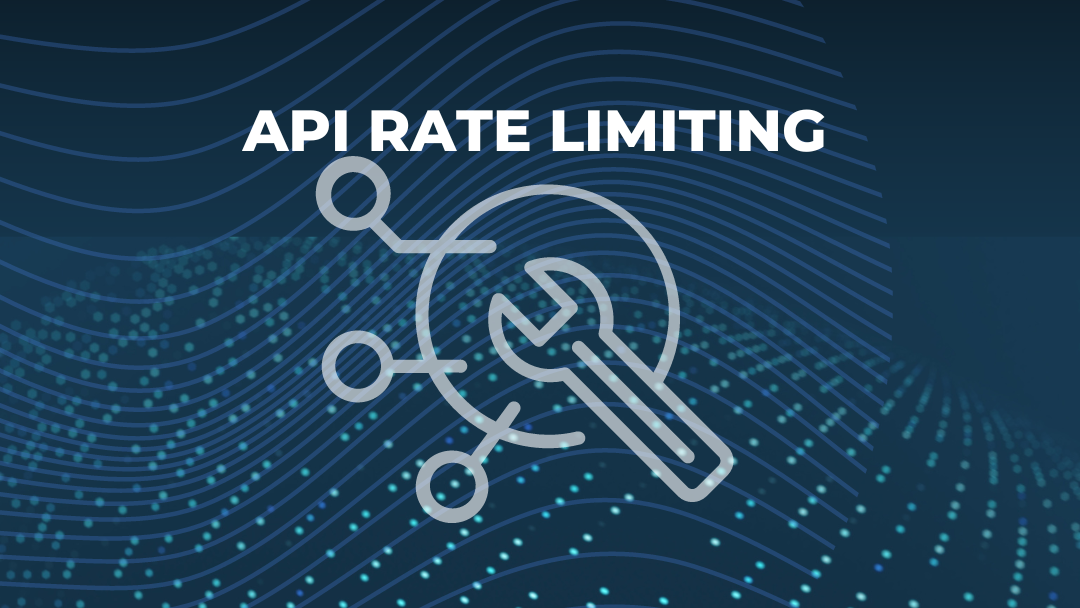
Introduction
As the world continues to embrace the digital revolution, Application Programming Interfaces (APIs) have become instrumental in enabling seamless interaction between different software applications. APIs enable developers to harness the functionalities of other software applications without necessarily understanding their internal workings. However, this does not mean that APIs can be used recklessly. APIs are resources that need to be protected, and one key aspect of this protection is API rate limiting.
The Essence of API Rate Limiting
At its core, API rate limiting is a technique used to control the number of requests a client can send to a server in a given period. Without rate limiting, APIs are prone to abuse, such as resource hogging by some clients at the expense of others, denial-of-service attacks, and potentially causing servers to crash.
Rate limiting helps ensure fair usage by preventing any single user or client from monopolizing the server’s resources. This not only makes the service available to as many users as possible, but it also helps maintain the overall health and performance of the API by reducing the likelihood of it becoming overwhelmed with requests.
Different Types of Rate Limiting
Fixed Window Rate Limiting
This technique allocates a maximum number of requests that a client can make within a fixed time window, such as an hour or a day. Once the limit is reached, the client must wait until the next time window begins.
Sliding Log Rate Limiting
Sliding log rate limiting is more dynamic. It allows the server to check the number of requests made in the current window and the preceding ones. This is more flexible as it can stop an unusually high rate of requests even if the overall limit for the current window hasn’t been reached.
Token Bucket Rate Limiting
This technique gives a client a number of tokens, each representing a request. The tokens are replenished at a fixed rate. When a client makes a request, they spend a token. If a client exhausts all tokens, they must wait for them to be replenished.
Implementing API Rate Limiting
The implementation of API rate limiting varies from one context to another. It’s dependent on factors like the specific demands of your API, your server infrastructure, and the expected behavior of your clients. However, some fundamental steps will help you implement API rate limiting efficiently.
Determine Your Rate Limits
You need to establish the number of requests a client can make within a specific time. This involves understanding your API’s capacity and balancing it against fair usage for your clients.
Communicate Your Rate Limits
It’s important to clearly communicate your rate limits to your clients. You can do this via HTTP headers that inform clients of their current rate limit status.
Handle Limit Exceeding Gracefully
In the event a client exceeds their limit, ensure the API responds with a suitable HTTP status code (typically 429 – Too Many Requests) and a meaningful error message to let the client know they’ve exceeded their limit.
Example Implementation
This is an example of rate limiting using the fixed-window strategy in an Express.js API with Node.js and Redis.
We’re going to use Redis to store our request count for each client within a fixed window. We will identify each client using their IP address.
Firstly, ensure you have the necessary packages installed:
npm install express redis moment
Here’s the code:
const express = require('express');
const redis = require('redis');
const moment = require('moment');
const app = express();
const client = redis.createClient();
app.use((req, res, next) => {
const ip = req.ip;
const now = moment().unix(); // current timestamp in seconds
const window = 3600; // 1 hour in seconds
const limit = 1000; // request limit per window
client.multi()
.set([ip, 0, 'EX', window, 'NX']) // Set IP with a value of 0, only if it doesn't exist already
.incr(ip) // Increment the value of IP
.exec((err, replies) => {
if (err) {
return res.status(500).send(err.message);
}
const requestCount = replies[1];
if (requestCount > limit) {
return res.status(429).send('Rate limit exceeded. Try again in an hour.');
}
res.set({
'X-RateLimit-Limit': limit,
'X-RateLimit-Remaining': limit - requestCount,
});
next();
});
});
app.get('/', (req, res) => {
res.send('Hello, you have not exceeded the rate limit!');
});
app.listen(3000);
In this implementation, every incoming request triggers middleware that checks the rate limit. If the IP doesn’t exist in the Redis store (first request from this IP in the current window), it’s set with a value of 0 and an expiry equal to the window size. If the IP does exist, the value (request count) is incremented. If the request count exceeds the limit, the API responds with a 429 status code. If not, it allows the request and sends rate limit info in the response headers.
The Role of API Management Solutions
API management solutions offer an all-in-one suite to manage, monitor, and secure APIs. These tools typically include built-in features for implementing API rate limiting, allowing you to control the usage of your APIs effectively without the need to code this functionality from scratch. Some popular API management tools include Apigee, Kong, and AWS API Gateway.
Conclusion
API rate limiting is a vital part of any API management strategy. It ensures that your APIs remain healthy, available, and resilient against potential abuse or overuse. While it may add an extra layer of complexity to your API management, the long-term benefits it provides in terms of reliability and stability far outweigh the initial effort.